Identify if swift code has syntax error
using AI
Below is a free classifier to identify if swift code has syntax error. Just input your text, and our AI will predict if there's a syntax error in the Swift code - in just seconds.
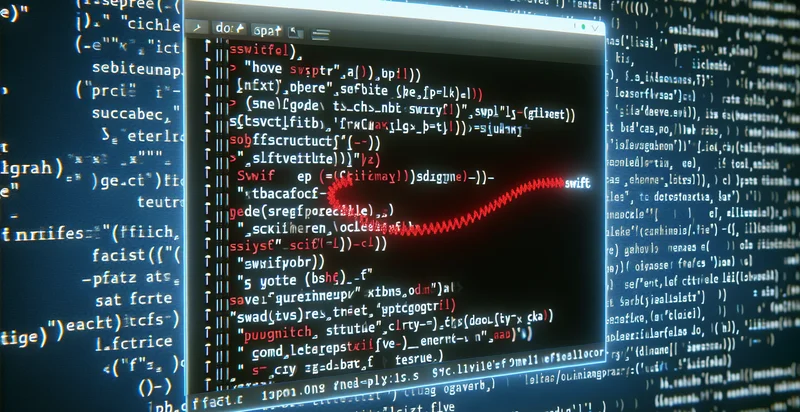
API Access
import nyckel
credentials = nyckel.Credentials("YOUR_CLIENT_ID", "YOUR_CLIENT_SECRET")
nyckel.invoke("if-swift-code-has-syntax-error", "your_text_here", credentials)
fetch('https://www.nyckel.com/v1/functions/if-swift-code-has-syntax-error/invoke', {
method: 'POST',
headers: {
'Authorization': 'Bearer ' + 'YOUR_BEARER_TOKEN',
'Content-Type': 'application/json',
},
body: JSON.stringify(
{"data": "your_text_here"}
)
})
.then(response => response.json())
.then(data => console.log(data));
curl -X POST \
-H "Content-Type: application/json" \
-H "Authorization: Bearer YOUR_BEARER_TOKEN" \
-d '{"data": "your_text_here"}' \
https://www.nyckel.com/v1/functions/if-swift-code-has-syntax-error/invoke
How this classifier works
To start, input the text that you'd like analyzed. Our AI tool will then predict if there's a syntax error in the Swift code.
This pretrained text model uses a Nyckel-created dataset and has 2 labels, including Has Syntax Error and No Syntax Error.
We'll also show a confidence score (the higher the number, the more confident the AI model is around if there's a syntax error in the Swift code).
Whether you're just curious or building if swift code has syntax error detection into your application, we hope our classifier proves helpful.
Recommended Classifiers
Need to identify if swift code has syntax error at scale?
Get API or Zapier access to this classifier for free. It's perfect for:
- Syntax Error Detection in Development: This use case involves using the text classification function during software development to automatically identify syntax errors in Swift code. Developers can receive immediate feedback on their code, streamlining the debugging process and reducing the time spent on error correction.
- Continuous Integration/Continuous Deployment (CI/CD) Pipeline: The function can be integrated into a CI/CD pipeline to analyze Swift code before deployment. By catching syntax errors early, teams can ensure that only well-formed code is pushed to production, minimizing the risk of runtime failures.
- Automated Code Review: Implement the identifier in an automated code review tool to flag syntax errors in pull requests. This enhances code quality by allowing developers to quickly address issues before the code is merged, fostering better collaboration and reducing manual review workloads.
- Educational Tools for Swift Programming: Universities and online learning platforms can utilize the function in educational environments to assist students in learning Swift. By providing real-time feedback on syntax errors, students can understand their mistakes immediately and improve their coding skills effectively.
- Code Quality Assurance: The function can be used by quality assurance teams to validate Swift code in applications before it undergoes further testing. By ensuring syntax correctness, QA personnel can focus on functional and performance testing, leading to a more robust software product.
- Integrated Development Environment (IDE) Enhancements: Enhance IDEs with this text classification function to provide developers with instant syntax error detection. This feature can improve user experience by offering suggestions or solutions, thereby increasing efficiency and reducing frustration for developers.
- Legacy Code Migration and Refactoring: During the migration of legacy systems to modern Swift code, the function can help identify and correct syntax errors in existing codebases. This ensures a smoother transition to modern practices while maintaining the integrity of the original functionality, ultimately leading to improved maintainability.