Identify if private key is in api responses
using AI
Below is a free classifier to identify if private key is in api responses. Just input your text, and our AI will predict if the private key is present - in just seconds.
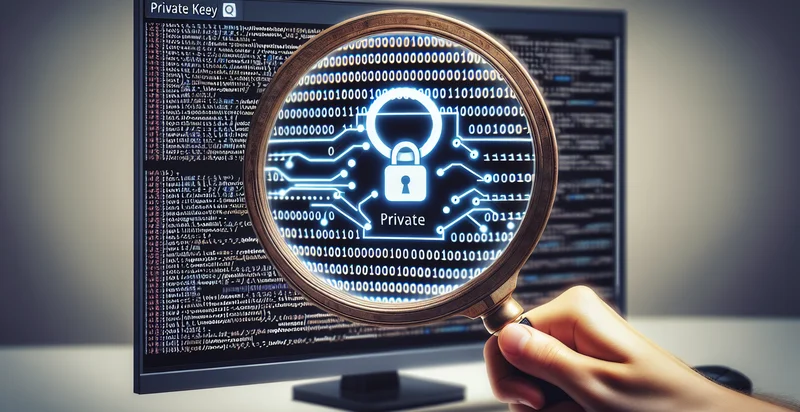
Contact us for API access
Or, use Nyckel to build highly-accurate custom classifiers in just minutes. No PhD required.
Get started
import nyckel
credentials = nyckel.Credentials("YOUR_CLIENT_ID", "YOUR_CLIENT_SECRET")
nyckel.invoke("if-private-key-is-in-api-responses", "your_text_here", credentials)
fetch('https://www.nyckel.com/v1/functions/if-private-key-is-in-api-responses/invoke', {
method: 'POST',
headers: {
'Authorization': 'Bearer ' + 'YOUR_BEARER_TOKEN',
'Content-Type': 'application/json',
},
body: JSON.stringify(
{"data": "your_text_here"}
)
})
.then(response => response.json())
.then(data => console.log(data));
curl -X POST \
-H "Content-Type: application/json" \
-H "Authorization: Bearer YOUR_BEARER_TOKEN" \
-d '{"data": "your_text_here"}' \
https://www.nyckel.com/v1/functions/if-private-key-is-in-api-responses/invoke
How this classifier works
To start, input the text that you'd like analyzed. Our AI tool will then predict if the private key is present.
This pretrained text model uses a Nyckel-created dataset and has 2 labels, including Private Key Found and Private Key Not Found.
We'll also show a confidence score (the higher the number, the more confident the AI model is around if the private key is present).
Whether you're just curious or building if private key is in api responses detection into your application, we hope our classifier proves helpful.
Recommended Classifiers
Need to identify if private key is in api responses at scale?
Get API or Zapier access to this classifier for free. It's perfect for:
- API Security Auditing: Implement the True text classification function to automatically scan API responses for any private keys. This helps security teams identify potential exposure of sensitive information, ensuring compliance with security policies and reducing the risk of data breaches.
- Continuous Integration/Continuous Deployment (CI/CD) Pipelines: Integrate the text classification function into CI/CD pipelines to validate that API responses do not leak private keys during automated testing. This enhances security during deployment by ensuring that no hard-coded secrets are pushed to production environments.
- Incident Response: Utilize the classification function during security incident investigations to analyze API logs for any instances of leaked private keys. Quick identification of these leaks can enable faster mitigation and a more robust incident response plan.
- Third-Party API Integration Monitoring: Deploy the function to monitor responses from third-party APIs that your application relies on. By ensuring that no private keys are present in the responses, organizations can minimize vulnerabilities associated with external services.
- Data Privacy Compliance: Use the text classification feature to ensure compliance with data protection regulations by checking that private keys are not included in returned data. This proactively addresses legal requirements related to sensitive information handling.
- Code Review Assistance: Incorporate the classification function in code review tools to automatically flag instances where API responses might include private keys. This helps developers maintain coding best practices and reinforces security awareness during the development process.
- Threat Intelligence Gathering: Employ the function to analyze API response patterns for signs of malicious activity attempting to extract private keys. This can inform threat intelligence efforts, helping organizations adapt defensive strategies against potential attacks targeting sensitive information.