Identify if oauth token is in source code
using AI
Below is a free classifier to identify if oauth token is in source code. Just input your text, and our AI will predict if an OAuth token is present in the source code - in just seconds.
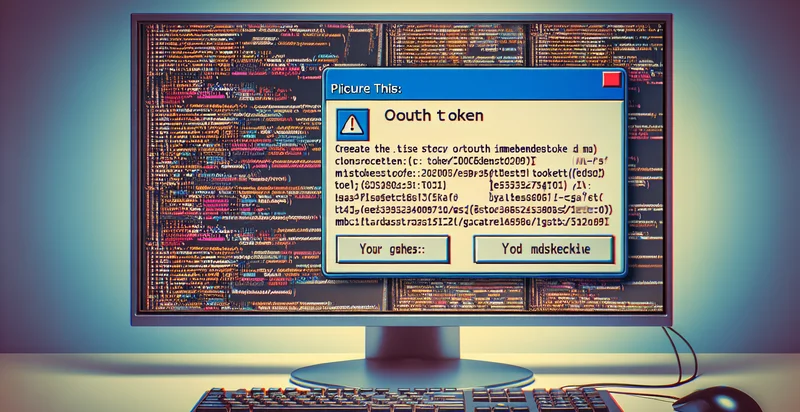
Contact us for API access
Or, use Nyckel to build highly-accurate custom classifiers in just minutes. No PhD required.
Get started
import nyckel
credentials = nyckel.Credentials("YOUR_CLIENT_ID", "YOUR_CLIENT_SECRET")
nyckel.invoke("if-oauth-token-is-in-source-code", "your_text_here", credentials)
fetch('https://www.nyckel.com/v1/functions/if-oauth-token-is-in-source-code/invoke', {
method: 'POST',
headers: {
'Authorization': 'Bearer ' + 'YOUR_BEARER_TOKEN',
'Content-Type': 'application/json',
},
body: JSON.stringify(
{"data": "your_text_here"}
)
})
.then(response => response.json())
.then(data => console.log(data));
curl -X POST \
-H "Content-Type: application/json" \
-H "Authorization: Bearer YOUR_BEARER_TOKEN" \
-d '{"data": "your_text_here"}' \
https://www.nyckel.com/v1/functions/if-oauth-token-is-in-source-code/invoke
How this classifier works
To start, input the text that you'd like analyzed. Our AI tool will then predict if an OAuth token is present in the source code.
This pretrained text model uses a Nyckel-created dataset and has 2 labels, including Contains Oauth Token and Does Not Contain Oauth Token.
We'll also show a confidence score (the higher the number, the more confident the AI model is around if an OAuth token is present in the source code).
Whether you're just curious or building if oauth token is in source code detection into your application, we hope our classifier proves helpful.
Related Classifiers
Need to identify if oauth token is in source code at scale?
Get API or Zapier access to this classifier for free. It's perfect for:
- Source Code Security Audits: Implement the identifier as part of periodic security audits to automatically detect if OAuth tokens are inadvertently included in source code repositories. This proactive approach can mitigate risks associated with unauthorized access and data breaches.
- Continuous Integration/Continuous Deployment (CI/CD) Pipelines: Integrate the text classification function into CI/CD pipelines to evaluate code changes in real-time. By flagging any instances of OAuth tokens during code commits, teams can prevent sensitive information from being deployed to production environments.
- Developer Training and Awareness: Utilize the identifier as a teaching tool in developer training programs. By demonstrating how frequently OAuth tokens may be accidentally committed to code, organizations can raise awareness about security best practices and promote safer coding behaviors.
- Code Review Assistance: Enhance code review processes by incorporating the identifier in code review tools. Reviewers can receive automated alerts regarding the presence of OAuth tokens, allowing for quicker identification and resolution of potential security issues.
- Security Compliance and Auditing: Use the function in compliance checks to ensure that development teams adhere to security policies that prohibit the inclusion of sensitive tokens in source code. Automating this assessment can streamline audits and support compliance with industry standards.
- Incident Response and Remediation: Enable security teams to leverage the identifier during incident response investigations. When a breach occurs, the function can help trace back to code origins where OAuth tokens were exposed, aiding in remediation efforts.
- Integration with Static Application Security Testing (SAST) Tools: Incorporate the identifier into SAST tools to enhance the security analysis of applications. By automatically scanning for OAuth tokens, these tools can provide a more comprehensive evaluation of code vulnerabilities before application deployment.