Identify if assembly code has syntax error
using AI
Below is a free classifier to identify if assembly code has syntax error. Just input your text, and our AI will predict if there is a syntax error - in just seconds.
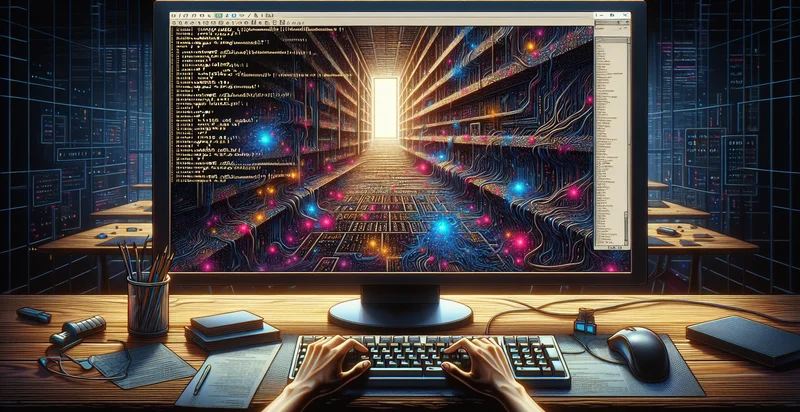
Contact us for API access
Or, use Nyckel to build highly-accurate custom classifiers in just minutes. No PhD required.
Get started
import nyckel
credentials = nyckel.Credentials("YOUR_CLIENT_ID", "YOUR_CLIENT_SECRET")
nyckel.invoke("if-assembly-code-has-syntax-error", "your_text_here", credentials)
fetch('https://www.nyckel.com/v1/functions/if-assembly-code-has-syntax-error/invoke', {
method: 'POST',
headers: {
'Authorization': 'Bearer ' + 'YOUR_BEARER_TOKEN',
'Content-Type': 'application/json',
},
body: JSON.stringify(
{"data": "your_text_here"}
)
})
.then(response => response.json())
.then(data => console.log(data));
curl -X POST \
-H "Content-Type: application/json" \
-H "Authorization: Bearer YOUR_BEARER_TOKEN" \
-d '{"data": "your_text_here"}' \
https://www.nyckel.com/v1/functions/if-assembly-code-has-syntax-error/invoke
How this classifier works
To start, input the text that you'd like analyzed. Our AI tool will then predict if there is a syntax error.
This pretrained text model uses a Nyckel-created dataset and has 2 labels, including Has Syntax Error and No Syntax Error.
We'll also show a confidence score (the higher the number, the more confident the AI model is around if there is a syntax error).
Whether you're just curious or building if assembly code has syntax error detection into your application, we hope our classifier proves helpful.
Related Classifiers
Need to identify if assembly code has syntax error at scale?
Get API or Zapier access to this classifier for free. It's perfect for:
- Automated Code Review: This function can be integrated into continuous integration/continuous deployment (CI/CD) pipelines to automatically check for syntax errors in assembly code during code review processes. Developers can receive immediate feedback, reducing the time spent on manual code inspection and increasing overall code quality.
- Real-time IDE Support: Incorporating this function into integrated development environments (IDEs) can provide real-time syntax checking for assembly code as developers type. This instant validation helps catch errors early, improving developer productivity and reducing debugging time.
- Educational Tools for Learners: This function can be utilized in educational software designed for teaching assembly language programming. It can provide students with instant feedback on their code submissions, enhancing their learning experience by allowing them to correct mistakes immediately.
- Code Refactoring Assistance: During the process of refactoring existing assembly code, this function can help identify syntax errors that may arise from changes made to the code. This support ensures that refactoring efforts do not introduce new bugs, maintaining the integrity of the codebase.
- Static Code Analysis Tools: The syntax error identifier can be a component in static code analysis tools specifically for assembly language. By automating the error-checking process, developers can efficiently analyze large codebases without manual inspection, ensuring higher compliance with coding standards.
- Legacy Code Maintenance: In environments where legacy assembly code is maintained, this function can help programmers identify and fix syntax errors that might have emerged after modifications. This is particularly valuable for teams unfamiliar with older syntax, ensuring the code remains functional and up-to-date.
- Error Reporting Systems: The function can be a feature within error reporting systems that log syntax errors encountered during assembly code compilation. By categorizing and returning specific error messages, it aids developers in quickly pinpointing issues that need to be resolved, streamlining the debugging process.